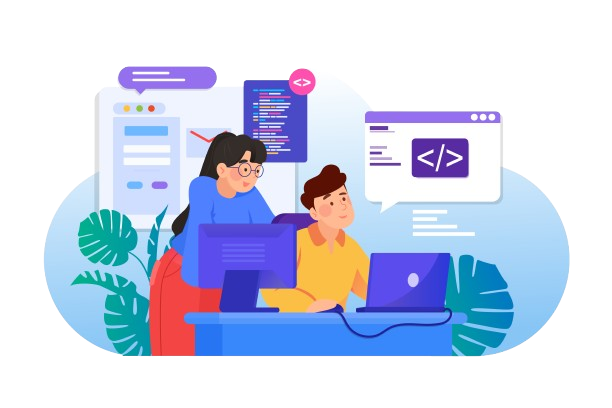
Standard 8 (Introduction to Programming)
- Basic Computer Literacy: Introduction to computers, operating systems, and basic computer operations.
- Introduction to Programming: Concepts of programming, what a program is, and how programming languages work.
- Scratch or Blockly: Visual programming languages to teach basic logic, sequencing, and problem-solving through fun projects.
- HTML & CSS Basics: Introduction to web development, creating simple static web pages.
- Basic Mathematics in Programming: Using simple arithmetic in programming to solve problems.
1. Basic Computer Literacy
Why Choose Us
WELL! Here Are Some Of Our Offerings...
Introduction to Computers
- Understanding what a computer is, the different types (desktop, laptop, tablets), and their primary components (CPU, RAM, storage, input/output devices).
- Overview of how computers are used in daily life, education, business, etc.
Operating Systems
- Introduction to operating systems (Windows, macOS, Linux).
- Basic operations like booting up, shutting down, installing software, and file management.
- Understanding the user interface: desktop, taskbar, file explorer, settings.
Basic Computer Operations
- How to create, open, save, and manage files and folders.
- Introduction to keyboard shortcuts, basic typing skills, and using a mouse or touchpad.
- Understanding file types (documents, images, videos, etc.) and software applications (Word processors, web browsers).
2. Introduction to Programming
Why Choose Us
WELL! Here Are Some Of Our Offerings...
Concepts of Programming
- What is programming? Explanation of how programming allows humans to communicate with computers.
- Understanding algorithms as a step-by-step set of instructions to solve a problem.
- Introduction to different programming languages (e.g., Python, JavaScript) and why multiple languages exist.
What is a Program?
- Explanation of what a program is: a set of instructions written in a programming language to perform a specific task.
- Examples of programs students use daily (e.g., apps, games, websites) and how these are all made using programming.
How Programming Languages Work
- Understanding the basics of how a programming language translates human instructions into machine code that the computer can execute.
- Explanation of the programming process: writing code, compiling or interpreting, debugging, and running the program.
3. Scratch or Blockly
Visual Programming Languages
- Introduction to Scratch or Blockly, which are block-based visual programming languages designed to make programming easy and fun.
- Explanation of how visual programming works: instead of typing code, you drag and drop blocks that represent different programming commands.
Teaching Basic Logic
- Using Scratch/Blockly to teach basic programming logic like sequencing (the order in which instructions are executed), loops (repeating a set of instructions), and conditionals (making decisions based on certain conditions).
- Simple exercises, like creating a character that moves on the screen based on commands, to demonstrate how logic is implemented in programming.
Sequencing and Problem-Solving
- Introduction to the concept of sequencing and how the order of commands affects the outcome.
- Problem-solving exercises where students create simple programs to solve puzzles or complete tasks.
Fun Projects
- Creating fun projects like animations, games, and interactive stories that reinforce programming concepts while keeping students engaged.
4. HTML & CSS Basics
Introduction to Web Development
- What is a website? Explanation of how websites work, the difference between static and dynamic websites.
- Overview of web technologies: HTML (Hypertext Markup Language) for structure, CSS (Cascading Style Sheets) for styling, and JavaScript for interactivity.
HTML Basics
- Learning HTML tags and elements, understanding the structure of an HTML document.
- Creating basic web pages with headings, paragraphs, lists, links, and images.
- Introduction to semantic HTML: using the correct tags for the right purpose (e.g.,
,
CSS Basics
- Understanding how CSS is used to style HTML elements, including color, fonts, layout, and spacing.
- Introduction to CSS syntax: selectors, properties, and values.
- Creating simple styled web pages: applying colors, changing fonts, adding borders, and adjusting margins/padding.
Creating Simple Static Web Pages
- Hands-on projects where students build their own static web pages, combining HTML and CSS.
- Encouraging creativity by allowing students to design their own web pages, like a personal homepage or a simple portfolio.
5. Basic Mathematics in Programming
Using Arithmetic in Programming
- Teaching how basic arithmetic operations (addition, subtraction, multiplication, division) are used in programming.
- Examples of how these operations are applied in real-world programming tasks, like calculating scores in games or creating simple calculators.
Simple Problem-Solving
- Creating programs that solve basic mathematical problems, such as finding the average of numbers, converting units (e.g., kilometers to miles), or calculating the area of shapes.
- Introduction to loops and conditionals through math-related problems, like summing a series of numbers or finding the largest of a set.
Understanding Variables
- Introduction to variables: what they are, how they store data, and how they can be manipulated in a program.
- Basic exercises on assigning values to variables and using them in arithmetic operations.
Integrating Math with Programming Projects
- Encouraging students to integrate their math skills with programming projects, such as creating a simple budgeting tool or a math quiz game.
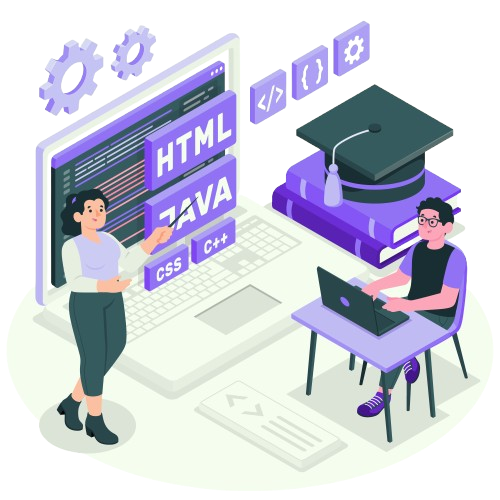
Standard 9 (Foundational Programming Skills)
- Introduction to Python: Basic syntax, variables, data types, input/output operations.
- Control Structures: Conditionals (if-else) and loops (for, while).
- Functions and Modules: Introduction to functions, defining functions, and using built-in modules.
- Introduction to Algorithms: Basic algorithms like sorting, searching, and their applications.
- Advanced HTML & CSS: Building more complex web pages with styling and layout techniques.
1. Introduction to Python
Basic Syntax
- Introduction to Python as a beginner-friendly programming language.
- Understanding Python syntax and structure: how to write and run a simple Python program.
- Explanation of indentation in Python and its significance.
Variables and Data Types
- Deeper understanding of variables: assigning values, naming conventions, and best practices.
- Introduction to different data types in Python: integers, floats, strings, booleans.
- Performing operations on different data types and understanding type conversion.
Input/Output Operations
- Using the input() function to get user input in Python programs.
- Introduction to the print() function for displaying output.
- Creating simple interactive programs that take user input and produce output based on it.
2. Control Structures
Conditionals (if-else)
- Introduction to decision-making in programming using if-else statements.
- Writing programs that make decisions based on user input or other conditions
- Understanding and implementing nested if-else statements.
Loops (for, while)
- Introduction to loops as a way to repeat a set of instructions multiple times.
- Understanding the difference between for loops and while loops.
- Practical examples: iterating over a range of numbers, repeating tasks until a condition is met.
- Nested loops: using loops inside other loops and understanding their applications.
Control Flow
- Understanding the flow of a program and how control structures like if-else and loops influence it.
- Using break and continue statements to control the flow within loops.
3. Functions and Modules
Introduction to Functions
- What is a function? Understanding the purpose of functions in programming.
- Creating and calling functions in Python.
- Understanding parameters and return values in functions.
Defining Functions
- Writing functions that perform specific tasks, like mathematical operations, string manipulation, etc.
- Understanding scope: local and global variables.
- Importance of code reusability and how functions help achieve it.
Control FlowUsing Built-in Modules
- Introduction to Python’s standard library and how to import and use modules.
- Practical examples: using the math module for advanced mathematical operations, random for generating random numbers.
- Exploring other useful modules like datetime, os, and sys.
4. Introduction to Algorithms
Basic Algorithms
- Introduction to what an algorithm is: a step-by-step procedure to solve a problem.
- Understanding the importance of algorithms in programming and problem-solving.
Sorting Algorithms
- Introduction to basic sorting algorithms like bubble sort and selection sort.
- Writing simple Python programs to implement these sorting algorithms.
- Understanding the concept of algorithm efficiency and time complexity (basic introduction to Big-O notation).
Searching Algorithms
- Introduction to searching algorithms: linear search and binary search.
- Writing Python programs to implement searching algorithms.
- Practical applications of sorting and searching in real-world scenarios.
5. Advanced HTML & CSS
Building More Complex Web Pages
- Introduction to more advanced HTML tags: forms, tables, and multimedia elements.
- Understanding how to create and style forms for user input.
Responsive Design
- Introduction to the concept of responsive design: making web pages that work well on different devices and screen sizes.
- Using media queries to create responsive layouts.
- Practical exercises in creating web pages that adapt to different screen sizes.
Styling with CSS
- Deeper understanding of CSS: applying styles using classes and IDs, grouping and nesting selectors.
- Introduction to CSS layout techniques: using Flexbox and Grid for responsive design.
- Creating visually appealing web pages by applying advanced styling techniques.
6. Project-Based Learning
Integration of Concepts
- Encouraging students to integrate Python programming, algorithms, and web development in small projects.
- Example projects:
- A simple quiz game that asks questions, checks answers, and keeps score.
- A personal blog website where the student creates a homepage, an about page, and a blog post page.
- A basic calculator or unit converter using Python functions.
- A portfolio website showcasing the student’s projects and skills.
Collaboration and Presentation
- Students can work in small groups on projects to encourage collaboration and peer learning.
- Regular presentations where students showcase their projects, explain the code, and discuss any challenges faced.
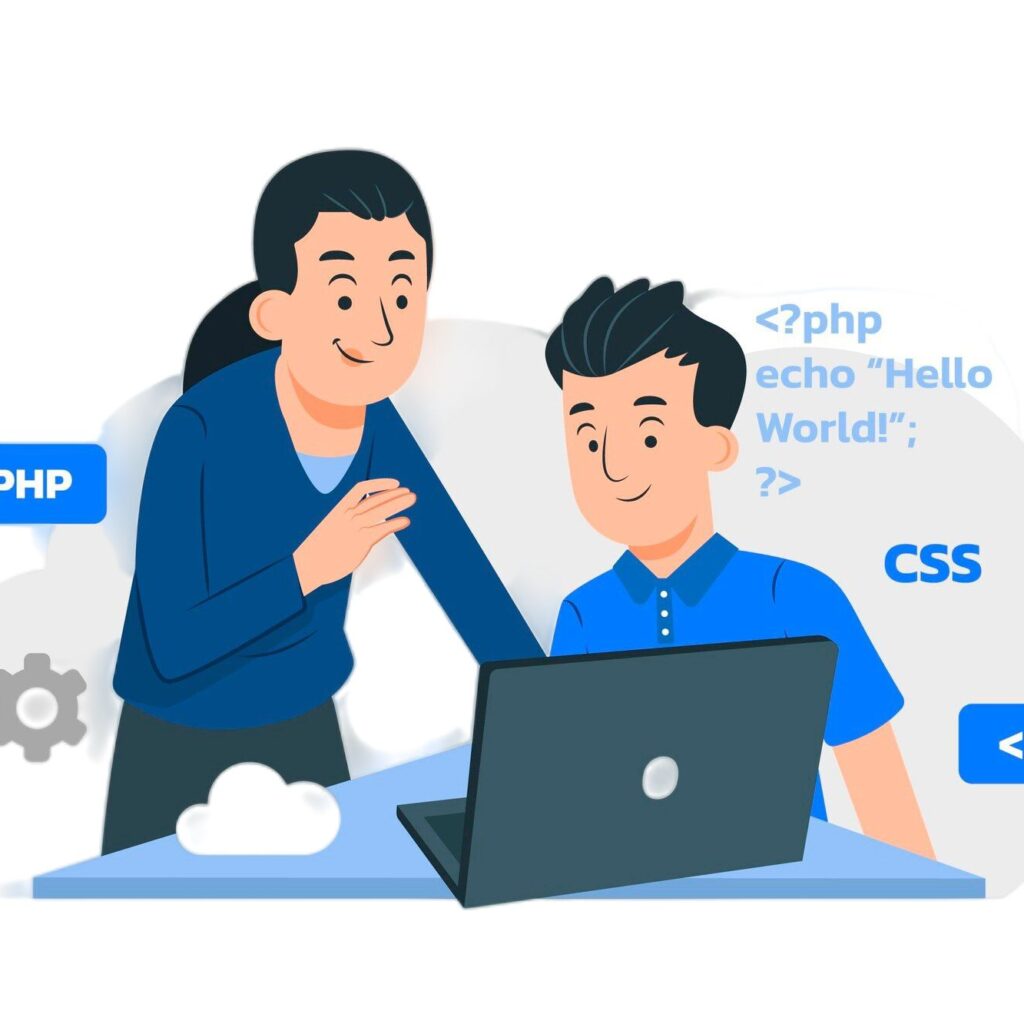
Standard 10 (Intermediate Programming Concepts)
- Object-Oriented Programming (OOP) in Python: Classes, objects, inheritance, polymorphism.
- Data Structures: Introduction to lists, dictionaries, stacks, queues, and their applications.
- Introduction to JavaScript: Basic syntax, DOM manipulation, and creating interactive web pages.
- Algorithmic Thinking: Problem-solving techniques, introduction to algorithms like bubble sort, selection sort, etc.
- Project-Based Learning: Creating small projects like a calculator, simple games, or a dynamic website.
1. Object-Oriented Programming (OOP) in Python
Introduction to OOP Concepts
- Understanding the principles of Object-Oriented Programming: Encapsulation, Abstraction, Inheritance, and Polymorphism.
- Explanation of why OOP is used and its advantages in programming.
Classes and Objects
- Introduction to classes and objects: what they are and how they are used in Python.
- Creating classes with attributes (variables) and methods (functions).
- Understanding the concept of an object as an instance of a class.
Inheritance and Polymorphism
- Explanation of inheritance: how a class can inherit attributes and methods from another class.
- Practical examples: creating a base class and derived classes to demonstrate inheritance.
- Introduction to polymorphism: understanding how different classes can have methods with the same name but different implementations.
Encapsulation and Abstraction
- Understanding encapsulation: hiding the internal state of an object and requiring all interactions to be performed through methods.
- Explanation of abstraction: simplifying complex reality by modeling classes appropriate to the problem.
- Practical examples: creating classes that encapsulate data and provide public methods to interact with that data.
2. Data Structures
Lists and Dictionaries
- In-depth understanding of lists: creating, modifying, and using lists in Python.
- Introduction to list operations: slicing, indexing, appending, and removing elements.
- Understanding dictionaries: key-value pairs, creating dictionaries, accessing and modifying data.
Stacks and Queues
- Introduction to stacks: LIFO (Last In, First Out) data structure, understanding its operations like push, pop, peek.
- Implementing stacks in Python using lists.
- Introduction to queues: FIFO (First In, First Out) data structure, understanding operations like enqueue, dequeue.
- Implementing queues in Python using lists or collections.deque.
Linked Lists
- Introduction to linked lists: understanding the difference between arrays and linked lists.
- Explanation of nodes and pointers, creating a simple singly linked list in Python.
- Understanding operations on linked lists: insertion, deletion, traversal.
3. Introduction to JavaScript
Basic Syntax and Concepts
- Introduction to JavaScript as a scripting language primarily used for web development.
- Understanding the basics of JavaScript syntax, including variables, data types, and operators.
- Writing simple JavaScript programs that run in a browser.
DOM Manipulation
- Introduction to the Document Object Model (DOM) and how JavaScript interacts with HTML/CSS.
- Understanding how to select and manipulate HTML elements using JavaScript (e.g., changing text, style, and content).
- Practical examples: creating interactive web pages where elements respond to user actions like clicks or keypresses.
Event Handling
- Understanding the concept of events in web development (e.g., clicks, keypresses, mouse movements).
- Learning how to handle events in JavaScript using event listeners.
- Practical examples: creating dynamic web pages that respond to user input.
Functions in JavaScript
- Creating and using functions in JavaScript.
- Understanding function scope, closures, and the difference between function declarations and expressions.
- Writing JavaScript programs that use functions to organize and reuse code.
4. Algorithmic Thinking
Problem-Solving Techniques
- Introduction to structured problem-solving techniques like breaking down problems into smaller, manageable parts.
- Understanding how to approach programming challenges systematically.
Sorting Algorithms
- In-depth understanding of more complex sorting algorithms like merge sort and quicksort.
- Writing Python programs that implement these sorting algorithms.
- Introduction to the concept of recursion and its application in sorting algorithms.
Algorithm Efficiency
- Introduction to Big-O notation to understand and compare the efficiency of different algorithms.
- Practical exercises to analyze and improve the efficiency of algorithms.
5. Project-Based Learning
Creating Small Projects
- Encouraging students to apply their knowledge in creating small, meaningful projects.
- Example projects:
- A to-do list web app that allows users to add, edit, and delete tasks using HTML, CSS, and JavaScript.
- A Python-based contact management system that stores contact details and allows users to search, add, and delete contacts.
- A simple game like Tic-Tac-Toe or Hangman implemented in Python with a focus on using OOP concepts.
Peer Review and Feedback
- Students can present their projects to the class, receive feedback, and discuss the challenges they faced.
- Encouraging peer review to foster collaboration and improve communication skills.
Integration of Concepts
- Projects should integrate multiple concepts learned during the year, such as OOP, data structures, and web development.
- Example: A personal finance tracker that allows users to input expenses and income, categorizes them, and displays a summary (integrating Python, data structures, and web development).
6. Preparation for Further Studies
Introduction to Version Control
- Basic introduction to version control systems like Git.
- Understanding the importance of version control in programming and collaboration.
- Practical exercises: setting up a Git repository, making commits, and understanding branches.
Coding Best Practices
- Introduction to coding best practices, including writing clean and readable code, commenting, and following naming conventions.
- Understanding the importance of documentation and how to create simple documentation for projects.
Exploration of Future Learning Paths
- Encouraging students to explore areas of interest for further studies, such as data science, web development, mobile app development, or AI/ML.
- Providing resources and guidance on how to pursue these areas in higher education or through self-study.
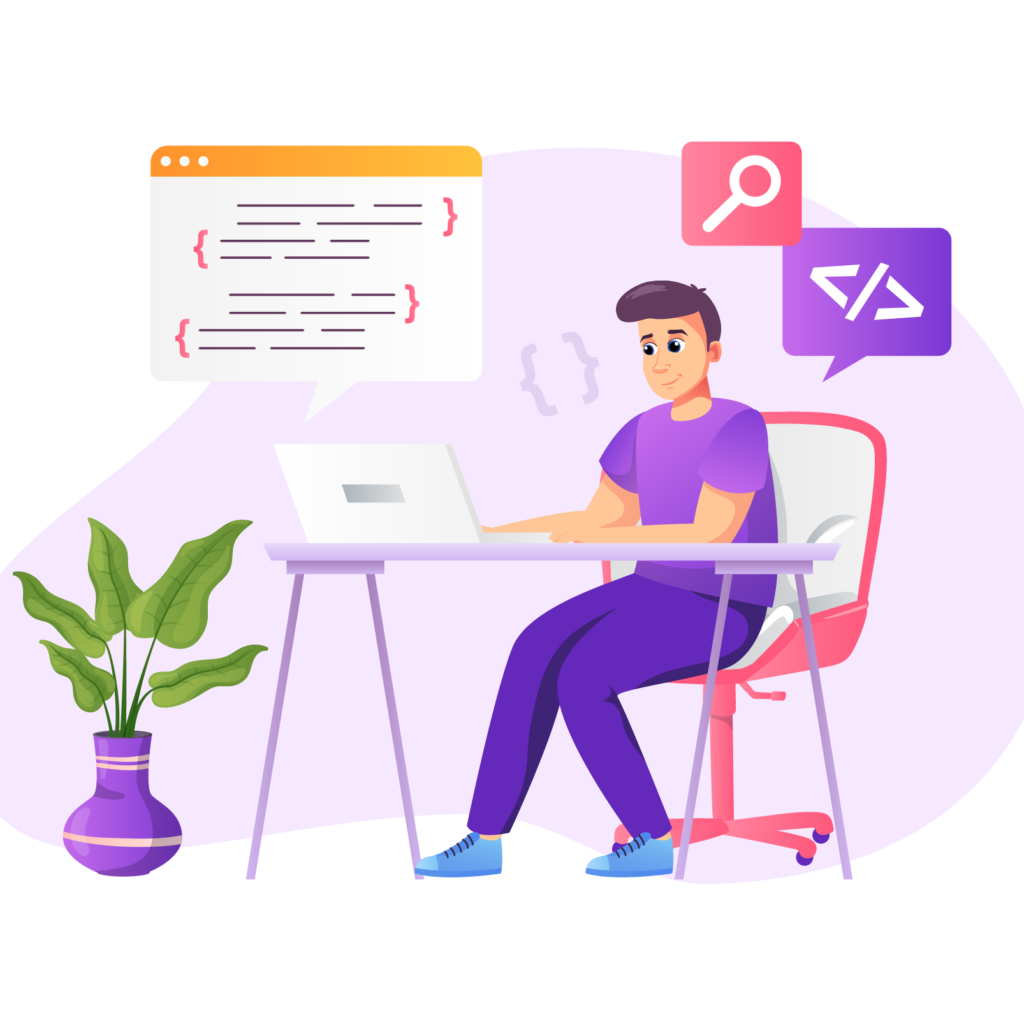
Standard 11 (Advanced Programming Concepts)
- Advanced Python Programming: File handling, exception handling, and advanced data structures like linked lists, trees.
- Web Development with JavaScript: Understanding the basics of web servers, client-server architecture, introduction to Node.js.
- Introduction to Databases: Basics of SQL, database design, and simple queries.
- Algorithms and Complexity: Understanding Big-O notation, more complex algorithms like merge sort, quicksort, etc.
- Project Work: Developing a full-fledged web application, integrating front-end and back-end.
1. Advanced Python Programming
File Handling
- Introduction to file handling in Python: understanding how to read from and write to files.
- Working with text files: opening, reading, writing, and closing files using open(), read(), write(), and close() methods.
- Practical exercises: creating programs that read from and write to files, like logging data or processing text files.
Advanced Data Structures
- Introduction to more complex data structures like sets, tuples, and dictionaries.
- Understanding when and how to use these data structures in Python.
- Introduction to more advanced topics like list comprehensions and generator expressions.
- Practical exercises: implementing algorithms that utilize these data structures efficiently.
Exception Handling
- Understanding the need for exception handling in programming: dealing with errors gracefully.
- Introduction to try-except blocks in Python: catching and handling exceptions.
- Using finally and else blocks to manage resources and execute code after exceptions are handled.
- Practical examples: handling user input errors, file handling errors, and implementing custom exceptions.
Linked Lists, Trees, and Graphs
- Deeper understanding of linked lists: singly and doubly linked lists.
- Introduction to trees: binary trees, binary search trees, and their operations (insertion, deletion, traversal).
- Basic understanding of graphs: representation of graphs using adjacency matrices and lists.
- Practical exercises: implementing and manipulating these data structures in Python.
2. Web Development with JavaScript
Introduction to Web Servers
- Understanding the basics of web servers: what they are and how they work.
- Introduction to client-server architecture: how data is transferred between the client (browser) and the server.
- Setting up a simple local server using Node.js and understanding the basics of HTTP requests and responses.
Introduction to Node.js
- Understanding what Node.js is: a runtime environment for executing JavaScript on the server-side.
- Basics of setting up a Node.js project: understanding npm (Node Package Manager), creating simple server-side scripts.
- Practical exercises: building a simple RESTful API using Node.js and Express.
Client-Server Communication
- Understanding the request-response cycle in web applications.
- Using JavaScript to send requests to a server and handle responses (e.g., using fetch() or XMLHttpRequest).
- Introduction to JSON (JavaScript Object Notation): how data is formatted and transferred between the client and server.
Dynamic Web Pages
- Introduction to creating dynamic web pages using JavaScript.
- Understanding the role of JavaScript in manipulating the DOM to create interactive and dynamic content.
- Practical examples: creating a dynamic to-do list, a simple interactive form, or a basic chat application.
3. Introduction to Databases
Basics of SQL
- Introduction to relational databases and SQL (Structured Query Language).
- Understanding database concepts: tables, rows, columns, primary keys, and foreign keys.
- Basic SQL operations: SELECT, INSERT, UPDATE, DELETE.
- Practical exercises: writing SQL queries to interact with a sample database.
Introduction to NoSQL Databases
- Basic understanding of NoSQL databases and their differences from relational databases.
- Overview of popular NoSQL databases like MongoDB, their use cases, and when to choose NoSQL over SQL.
- Practical examples: setting up a simple NoSQL database, storing and retrieving data using MongoDB.
Database Design
- Understanding the principles of database design: normalization, relationships, and data integrity.
- Designing a simple relational database schema for a real-world application (e.g., a student management system).
- Practical exercises: creating database schemas, designing tables, and defining relationships.
SQL vs NoSQL
- Understanding the key differences between SQL and NoSQL databases.
- Discussing use cases where each type of database is more appropriate.
- Practical exercises: comparing performance and use cases for SQL and NoSQL databases.
4. Algorithms and Complexity
Understanding Algorithm Complexity
- Introduction to Big-O notation: understanding how to measure the time and space complexity of algorithms.
- Comparing different algorithms based on their efficiency (e.g., comparing sorting algorithms like bubble sort, merge sort, and quicksort).
- Practical exercises: analyzing and optimizing algorithms for efficiency.
Graph Algorithms
- Introduction to basic graph algorithms like depth-first search (DFS) and breadth-first search (BFS).
- Understanding how graphs are used to model real-world problems like social networks, maps, and web page links.
- Practical exercises: implementing graph traversal algorithms and solving problems like finding the shortest path.
Recursive Algorithms
- Understanding the concept of recursion and how it is used to solve problems.
- Practical examples: writing recursive algorithms to solve problems like factorial calculation, Fibonacci sequence, and binary search.
- Discussing the trade-offs of using recursion, including stack depth and performance considerations.
Dynamic Programming
- Introduction to dynamic programming: understanding how to solve complex problems by breaking them down into simpler subproblems.
- Practical examples: solving problems like the knapsack problem, longest common subsequence, and coin change using dynamic programming.
- Discussing the advantages and challenges of dynamic programming, including memoization and tabulation techniques.
5. Project Work
Developing a Full-Fledged Web Application
- Encouraging students to apply their knowledge by developing a full-fledged web application that integrates front-end, back-end, and database components.
- Example projects:
- A blog platform with user authentication, post creation, and commenting system.
- A simple e-commerce site with product listings, shopping cart functionality, and checkout process.
- A student management system with a database to store student records and a web interface for CRUD operations.
Collaboration and Version Control
- Encouraging students to work in teams to foster collaboration and real-world experience.
- Using Git for version control: understanding branching, merging, and resolving conflicts.
- Practical exercises: collaborating on a project using GitHub or another version control platform, and presenting the project to the class.
Integration of Advanced Concepts
- Projects should integrate advanced concepts like algorithms, data structures, and databases.
- Example: A social networking site that includes features like user profiles, friend requests, messaging, and a feed that uses graph algorithms to suggest friends or content.
6. Preparation for Higher Education and Careers
Introduction to Software Development Methodologies
- Understanding different software development methodologies like Agile, Waterfall, and DevOps.
- Discussing the pros and cons of each methodology and where they are commonly used.
Exploration of Career Paths
- Discussing various career paths in computer science and technology, such as software development, data science, cybersecurity, and AI/ML.
- Providing resources and guidance on how to pursue these careers, including further education, online courses, and internships.
Coding Competitions and Hackathons
- Encouraging participation in coding competitions, hackathons, and other events to enhance problem-solving skills and gain exposure to real-world challenges.
- Providing tips and resources for preparing for these events, such as practicing coding challenges on platforms like HackerRank, LeetCode, or Codeforces.
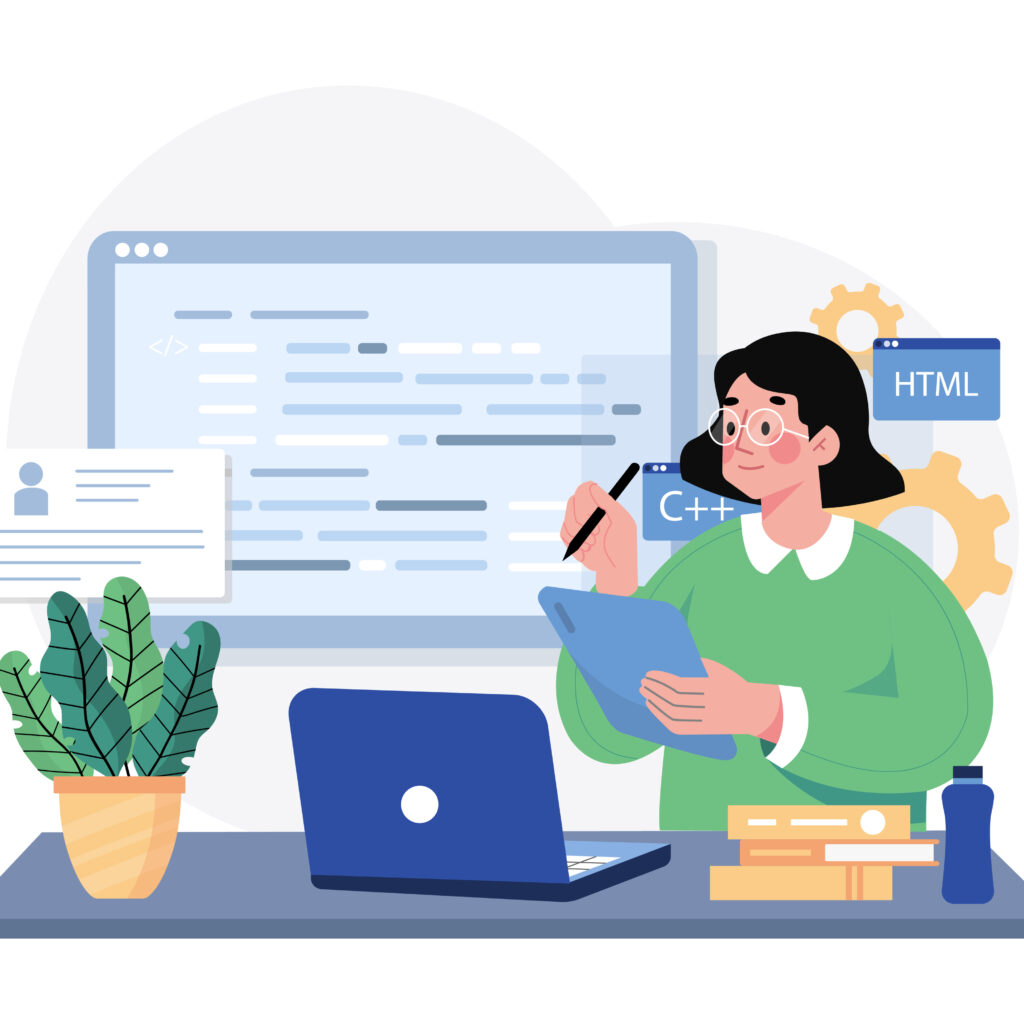
Standard 12 (Specialization and Applied Programming)
- Data Science Introduction: Basics of data science, data analysis with Python using libraries like Pandas and NumPy.
- Introduction to AI and Machine Learning: Basic concepts of AI/ML, simple machine learning models using Python.
- Advanced Web Development: Introduction to front-end frameworks like React.js, building single-page applications.
- Mobile App Development: Basics of mobile app development using React Native or Flutter.
- Capstone Project: A comprehensive project that integrates all the concepts learned, like creating a web application with a database, or a mobile app.
1. Data Science Introduction
Introduction to Data Science
- Understanding what data science is: the process of collecting, analyzing, and interpreting large sets of data to extract useful information.
- Overview of the data science pipeline: data collection, data cleaning, data analysis, and data visualization.
- Discussing the importance of data science in various industries like finance, healthcare, marketing, and technology.
Data Analysis with Python
- Introduction to libraries like Pandas and NumPy for data manipulation and analysis.
- Understanding how to work with datasets: loading, cleaning, transforming, and analyzing data using Python.
- Practical exercises: analyzing datasets to extract insights, such as calculating averages, finding correlations, and visualizing data with graphs and charts.
Introduction to Data Visualization
- Understanding the importance of data visualization in communicating data-driven insights.
- Introduction to libraries like Matplotlib and Seaborn for creating visualizations in Python.
- Practical examples: creating bar charts, line graphs, scatter plots, and heatmaps to represent data.
Case Studies and Real-World Applications
- Discussing case studies where data science has been used to solve real-world problems.
- Encouraging students to work on mini-projects where they apply data science techniques to analyze datasets and present their findings.
2. Introduction to AI and Machine Learning
Understanding AI and Machine Learning
- Introduction to Artificial Intelligence (AI) and Machine Learning (ML): understanding the difference between the two and their applications.
- Overview of how machines learn from data: supervised learning, unsupervised learning, and reinforcement learning.
Simple Machine Learning Models
- Introduction to basic machine learning algorithms: linear regression, decision trees, k-nearest neighbors.
- Understanding the concept of training and testing data, overfitting, and model evaluation.
- Practical examples: implementing simple machine learning models using Python libraries like Scikit-learn.
Exploring Neural Networks and Deep Learning
- Introduction to neural networks: understanding how they work and their role in deep learning.
- Overview of deep learning frameworks like TensorFlow and Keras.
- Practical exercises: building and training a basic neural network to solve a simple problem, like image classification or sentiment analysis.
AI Ethics and Impact
- Discussing the ethical considerations of AI and machine learning, including bias, privacy, and the societal impact of AI technologies.
- Encouraging students to think critically about the role of AI in society and the responsibilities of AI practitioners.
3. Advanced Web Development
Introduction to Front-End Frameworks
- Understanding the role of front-end frameworks in building modern web applications.
- Introduction to popular frameworks like React.js: understanding components, state, and props.
- Practical examples: building single-page applications (SPAs) using React.js or another front-end framework.
Building RESTful APIs
- Understanding RESTful APIs: what they are and how they enable communication between the front-end and back-end of a web application.
- Practical examples: designing and building RESTful APIs using Node.js and Express or Django.
- Integrating the front-end with the back-end: making API calls from the front-end to retrieve and display data.
Introduction to Full-Stack Development
- Understanding the concept of full-stack development: working with both the front-end and back-end of a web application.
- Practical examples: developing a full-stack web application that includes user authentication, data storage, and dynamic content.
- Introduction to deployment: deploying a web application to a cloud platform like Heroku, AWS, or Google Cloud.
Web Security Basics
- Introduction to web security concepts: understanding common vulnerabilities like SQL injection, XSS (Cross-Site Scripting), and CSRF (Cross-Site Request Forgery).
- Best practices for securing web applications: input validation, authentication, and encryption.
- Practical exercises: identifying and fixing security vulnerabilities in a web application.
4. Mobile App Development
Introduction to Mobile App Development
- Understanding the differences between mobile and web development: platform considerations, user interface design, and performance.
- Overview of popular mobile development frameworks like React Native or Flutter.
- Setting up a mobile development environment: building and running a simple mobile app on a simulator or device.
Building Cross-Platform Mobile Apps
- Introduction to cross-platform development: using frameworks like React Native or Flutter to build apps that work on both Android and iOS.
- Practical examples: building a simple mobile app that includes features like navigation, forms, and data storage.
- Understanding the challenges of mobile development: performance optimization, handling device-specific features, and ensuring a consistent user experience.
Deploying Mobile Apps
- Introduction to the app deployment process: preparing an app for submission to the Google Play Store and Apple App Store.
- Understanding app store guidelines and requirements: ensuring the app meets all necessary criteria for approval.
- Practical exercises: packaging and deploying a mobile app to the app stores, including generating APKs (Android) and IPAs (iOS).
5. Capstone Project
Developing a Comprehensive Project
- Encouraging students to apply all the skills they have learned by developing a comprehensive project that integrates multiple concepts.
- Example projects:
- A data-driven web application that uses machine learning to provide personalized recommendations.
- A mobile app that tracks and analyzes user activity, such as fitness tracking or budget management.
- A full-stack e-commerce platform with a user-friendly front-end, secure back-end, and payment integration.
Presentation and Evaluation
- Students present their capstone projects to the class or a panel of judges, explaining their design choices, demonstrating functionality, and discussing challenges faced.
- Projects are evaluated based on criteria like originality, complexity, usability, and adherence to best practices.
- Encouraging students to reflect on their learning experience and consider areas for further improvement.
Project Planning and Execution
- Teaching students how to plan and manage a software development project: defining requirements, setting milestones, and tracking progress.
- Emphasizing the importance of documentation: creating project documentation, including design documents, user manuals, and technical guides.
- Encouraging collaboration: working in teams, using version control (Git), and participating in code reviews.
6. Preparation for Higher Education and Careers
Exploring Specializations in Computer Science
- Discussing various specializations within computer science, such as cybersecurity, artificial intelligence, data science, and software engineering.
- Providing guidance on selecting a college major, applying to universities, and preparing for entrance exams like JEE, SAT, or other relevant tests.
Building a Professional Portfolio
- Encouraging students to build a portfolio that showcases their projects, skills, and achievements.
- Creating a personal website or GitHub repository where they can share their work with potential colleges or employers.
- Discussing the importance of networking, attending tech conferences, and participating in online communities.
Career Preparation
- Providing resources for preparing for technical interviews: practicing coding challenges, understanding common interview questions, and learning how to communicate effectively.
- Exploring internship opportunities: how to find internships, build a resume, and prepare for interviews.
- Discussing the importance of lifelong learning: staying current with new technologies, taking online courses, and pursuing certifications.
Full-Stack Development Study Plan
Week 1-2: Introduction to Web Development
Goals:
– Understand the basics of full-stack development and the roles of frontend and backend.Topics:
– Internet basics: How websites work
– Overview of frontend vs backend
– Setting up your development environment (VS Code, Git, Postman)Practice:
– Build a simple static website using HTML and CSS.
Week 5-6: Advanced JavaScript & ES6+
Goals:
– Learn modern JavaScript features and advanced concepts.Topics:
Practice:
– ES6+ features: arrow functions, destructuring, spread/rest operators
– Promises, async/await, and modules
– JavaScript best practices
– Refactor your previous projects using modern JavaScript features.
Week 3-4: HTML, CSS & JavaScript Basics
Goals:
– Gain proficiency in HTML, CSS, and basic JavaScript.Topics:
– HTML elements, attributes, and forms
– CSS selectors, box model, flexbox, and grid
– JavaScript basics: Variables, data types, operators, control flowPractice:
– Create a responsive multi-page website with interactive elements using JavaScript.
Week 7-8: Introduction to Version Control with Git
Goals:
– Understand version control and start using Git.Topics:
– Git basics: init, clone, add, commit, push, pull
– Branching and merging
– Collaborating with GitHubPractice:
– Push your previous projects to GitHub and collaborate with others.
Week 9-10: Introduction to Backend Development
Goals:
– Learn backend basics using Node.js (or another language of your choice).Topics:
– Setting up a basic HTTP server
– Introduction to RESTful APIs
– Handling requests and responsesPractice:
– Build a simple CRUD API using Node.js and Express.
Week 13-14: Frontend Frameworks (React)
Goals:
– Start building dynamic UIs using React.Topics:
– Component-based architecture
– JSX and Virtual DOM
– Props, state, and basic hooks (useState, useEffect)Practice:
– Build a to-do list app using React.
Week 11-12: Working with DatabasesGoals: - Understand how to interact with databases.
Topics:
– Introduction to SQL (MySQL/PostgreSQL) and NoSQL (MongoDB)
– Performing CRUD operations
– Database design and normalizationPractice:
– Connect your API to a database and perform CRUD operations.
Week 15-16: Advanced React & State Management
Goals:
– Deepen your understanding of React and state management.Topics:
– React Router for navigation
– Context API and state management
– Redux basicsPractice:
– Create a multi-page application with routing and state management.
Week 17-18: Backend Frameworks and Authentication
Goals:
– Implement a more robust backend with authentication.Topics:
– Middleware in Express (or your chosen framework)
– User authentication with JWT and sessions
– Role-based access control (RBAC)Practice:
– Add user authentication and authorization to your backend project.
Week 21-22: Testing (Frontend & Backend)
Goals:
– Implement testing for both frontend and backend.Topics:
– Unit testing with Jest (JavaScript)
– Testing React components with React Testing Library
– API testing with Postman or SupertestPractice:
– Write tests for your full-stack application to ensure functionality.
Week 19-20: Working with APIs and Integration
Goals:
– Learn to connect frontend with backend.Topics:
– Fetching data from your backend using Axios or Fetch API
– Error handling and data transformation
– Integrating third-party APIsPractice:
– Build a full-stack project that consumes your backend API and displays data on the frontend.
Week 23-24: Deployment & DevOps Basics
Goals:
– Learn how to deploy your full-stack application.Topics:
– Introduction to cloud services (AWS, Heroku, DigitalOcean)
– Setting up CI/CD pipelines (GitHub Actions)
– Docker basics for containerizationPractice:
– Deploy your full-stack application to a cloud service and set up a CI/CD pipeline.
Week 25-26: Building a Complete Full-Stack Project
Goals:
– Apply all your knowledge to build a complete full-stack application.Project Ideas:
– E-commerce platform with product management, shopping cart, and payment integration
– Social media platform with user profiles, posts, and comments
– Blogging platform with user authentication and content management
Continuous Learning & ResourcesAdditional Topics: - Microservices architecture - GraphQL - Advanced DevOps tools and practices
Useful Resources:
– Books:
– ‘The Pragmatic Programmer’ by Andrew Hunt and David Thomas
– ‘Clean Code’ by Robert C. Martin
– Websites:
– freeCodeCamp
– MDN Web DocsPractice Platforms:
– LeetCode
– HackerRank for full-stack challenges